What Is Iterate In Java? (2023)
Development Partners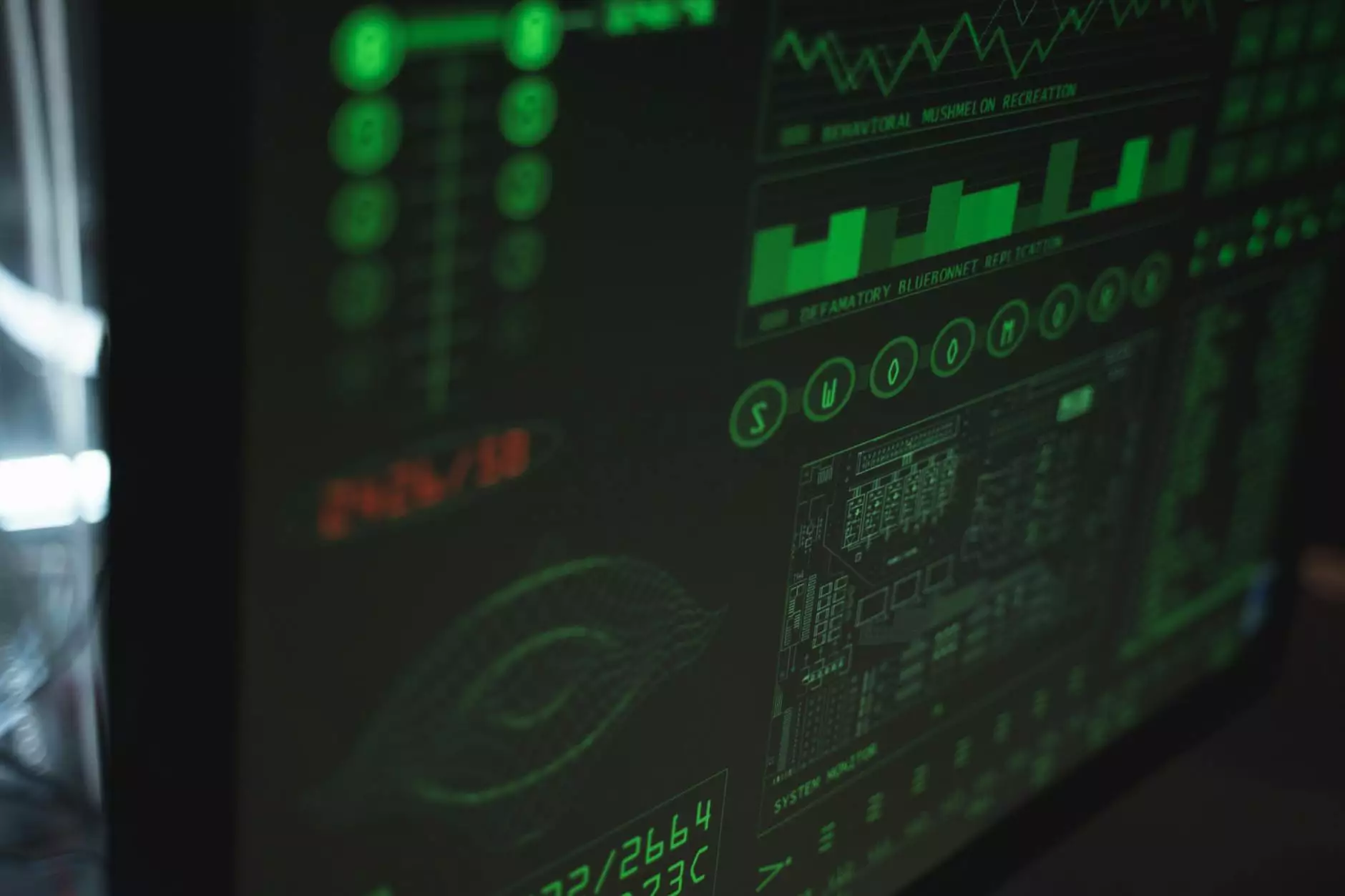
Introduction to Iteration in Java
When it comes to Java programming, iteration plays a crucial role in controlling the flow of execution and dealing with repetitive tasks efficiently. In this guide, Maslow Lumia Bartorillo Advertising provides a comprehensive overview of iterating in Java, covering various techniques and best practices to help you become a proficient Java developer.
Understanding Different Iteration Types
Java provides several ways to iterate over collections, arrays, and other data structures. Let's explore some of the most commonly used iteration types:
1. The for Loop
The for loop is a fundamental construct in Java, allowing you to repeat a block of code a specific number of times. Its syntax consists of an initialization statement, a condition, and an increment or decrement statement. This type of iteration is ideal when you know the exact number of iterations required.
2. The while Loop
The while loop continues to execute a block of code as long as a specific condition remains true. It is commonly used when the number of iterations is uncertain beforehand. You need to ensure that the condition eventually becomes false to avoid infinite loops.
3. The do-while Loop
The do-while loop is similar to the while loop, but it guarantees at least one execution of the block of code before evaluating the loop condition. This makes it useful when you want a code block to execute once, regardless of the initial condition.
4. The enhanced for Loop
The enhanced for loop, introduced in Java 5, simplifies iterating over collections and arrays. It provides a concise syntax for looping through elements without the need for an explicit index. This type of iteration is commonly used when you don't require access to the index or need to iterate over all the elements.
Advanced Iteration Techniques
Now that we have explored the basic iteration types, let's delve into advanced techniques that can enhance your Java programming skills:
1. Iterator Interface
The Iterator interface provides a universal way to traverse through collections. It provides methods such as hasNext() and next() to iterate over elements in a collection. This interface allows you to remove elements while iterating, which is not possible with basic for loops.
2. Iterable Interface
The Iterable interface is implemented by classes that define an iteration order. It enables the use of enhanced for loops to iterate over the objects of a class. By implementing this interface, you make your class iterable, allowing convenient iteration using for-each syntax.
3. Stream API
The Stream API, introduced in Java 8, provides functional-style operations to process collections and streams of data. It allows you to express complex iteration and transformation operations in a concise and readable manner using lambda expressions. Utilizing the Stream API can significantly enhance your code readability and maintainability.
4. Recursive Iteration
In some cases, recursive iteration can provide an elegant solution to repetitive tasks. It involves a method calling itself until a specific condition is met. Recursive iteration is particularly useful when dealing with complex data structures, such as trees and graphs.
Conclusion
Iterating in Java is a crucial skill for every developer. In this comprehensive guide, Maslow Lumia Bartorillo Advertising introduced you to different iteration types and advanced techniques available in Java. By mastering iteration, you can efficiently process and manipulate data, making your Java applications more powerful and efficient.
Remember, iteration is just one aspect of Java programming, and there are many other fundamental concepts to explore. Continuously expanding your knowledge and staying updated with the latest Java advancements will help you become a better programmer.